Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- react swiper
- web3-react
- 다중포인터
- nextjs
- 카카오지도 구현
- 메일 보내기 react
- Next.js 나이스 본인인증
- 프로그래머스
- use Client
- React 나이스 신원인증
- CSS
- nextjs contact us
- next15
- robots.txt
- App Router
- pass인증
- JavaScript
- 구글 메일보내기
- 알고리즘
- react
- swiper
- github
- nextjs 메일보내기
- 전체 너비로 css
- 프론트 본인인증
- 빈도수세기
- 사이트맵
- Til
- 카카오맵 api
- 함수
Archives
- Today
- Total
YEV.log
Next.js | Next.js + typescript 초기 세팅 (next 13) 본문
1. 설치 및 실행
설치
npx create-next-app --typescript
# or
yarn create next-app --typescript
# ...
What is your project named? (프로젝트 이름)
Would you like to use ESLint with this project? (eslint를 쓸건지 => yes)
실행
# 개발자 모드
npm run dev
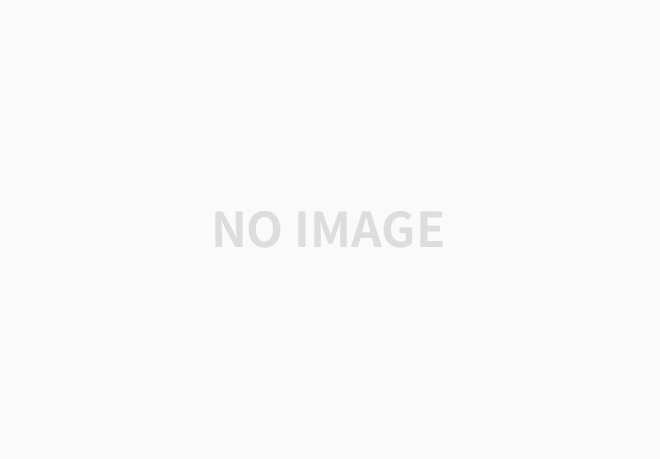
2. ESLint, Prettier 세팅
1) 설치
npm install --save-dev prettier eslint-config-prettier eslint-plugin-prettier
eslint-config-prettier : prettier와 충돌할 수 있는 eslint 규칙들을 꺼줍니다.
eslint-plugin-prettier : prettier가 eslint의 규칙으로써 동작하게 만들어줍니다.
2) .eslintrc.json 파일 삭제
3-1) .eslintrc 생성
{
"env": {
// 전역 변수 사용을 정의합니다. 추가하지 않으면 ESLint 규칙에 걸리게 됩니다.
"browser": true,
"es6": true,
"node": true
},
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended", // 해당 플러그인의 권장 규칙을 사용합니다.
"plugin:prettier/recommended" // plugin과 eslint-config-prettier 설정을 한번에 합니다.
],
"parser": "@typescript-eslint/parser", // ESLint 파서를 지정합니다.
"parserOptions": {
"ecmaFeatures": {
"jsx": true // JSX를 파싱할 수 있습니다.
},
"ecmaVersion": 12, // Modern ECMAScript를 파싱할 수 있습니다.
"sourceType": "module" // import, export를 사용할 수 있습니다.
},
"plugins": ["react", "@typescript-eslint"],
"rules": {
"no-var": "warn", // var 금지
"no-multiple-empty-lines": "warn", // 여러 줄 공백 금지
"no-nested-ternary": "warn", // 중첩 삼항 연산자 금지
"no-console": "warn", // console.log() 금지
"eqeqeq": "warn", // 일치 연산자 사용 필수
"dot-notation": "warn", // 가능하다면 dot notation 사용
"no-unused-vars": "warn", // 사용하지 않는 변수 금지
"react/destructuring-assignment": "warn", // state, prop 등에 구조분해 할당 적용
"react/jsx-pascal-case": "warn", // 컴포넌트 이름은 PascalCase로
"react/no-direct-mutation-state": "warn", // state 직접 수정 금지
"react/jsx-no-useless-fragment": "warn", // 불필요한 fragment 금지
"react/no-unused-state": "warn", // 사용되지 않는 state
"react/jsx-key": "warn", // 반복문으로 생성하는 요소에 key 강제
"react/self-closing-comp": "warn", // 셀프 클로징 태그 가능하면 적용
"react/jsx-curly-brace-presence": "warn", // jsx 내 불필요한 중괄호 금지
"prettier/prettier": [
"error",
{
"endOfLine": "auto"
}
]
},
"settings": {
"react": {
"version": "detect" // 현재 사용하고 있는 react 버전을 eslint-plugin-react가 자동으로 감지합니다.
}
}
}
3-2) .prettierrc 생성
{
"tabWidth": 2,
"endOfLine": "lf",
"arrowParens": "avoid",
"singleQuote": true
}
3. 폴더구조
저는 pages, styles, components 같은 파일들을 모두 src 폴더안에서 관리하고자 했습니다. 또한 불필요한 소스들을 삭제합니다.
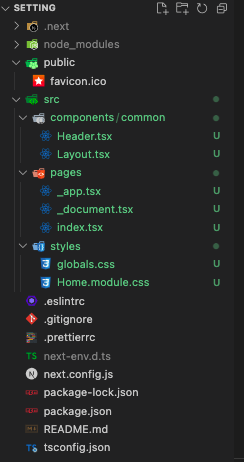
1) src > components > common
// Header.tsx
export default function Header() {
return (
<header
style={{
display: 'flex',
padding: '0 15px',
alignItems: 'center',
width: '100vw',
height: '60px',
fontSize: '30px',
backgroundColor: 'navy',
}}
>
❄️
</header>
);
}
// Layout.tsx
import Header from './Header';
interface Props {
children: React.ReactNode;
}
export default function Layout({ children }: Props) {
return (
<>
<Header />
{children}
</>
);
}
2) src > pages
// index.tsx
import Head from 'next/head';
import styles from '../styles/Home.module.css';
export default function Home() {
return (
<>
<Head>
<title>Create Next App</title>
<meta name="description" content="Generated by create next app" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="icon" href="/favicon.ico" />
</Head>
<main className={styles.main}>main page</main>
</>
);
}
// _document.tsx
import { Html, Head, Main, NextScript } from 'next/document'
export default function Document() {
return (
<Html lang="en">
<Head />
<body>
<Main />
<NextScript />
</body>
</Html>
)
}
// _app.tsx
import '../styles/globals.css';
import type { AppProps } from 'next/app';
import Layout from '../components/Layout';
export default function App({ Component, pageProps }: AppProps) {
return (
<Layout>
<Component {...pageProps} />
</Layout>
);
}
📌 _app.tsx 에서는 추후 Provider나, Style 관련 컴포넌트를 한눈에 보고, Layout 컴포넌트에서는 header와 footer를 한눈에 볼 수 있도록 분리하였습니다.
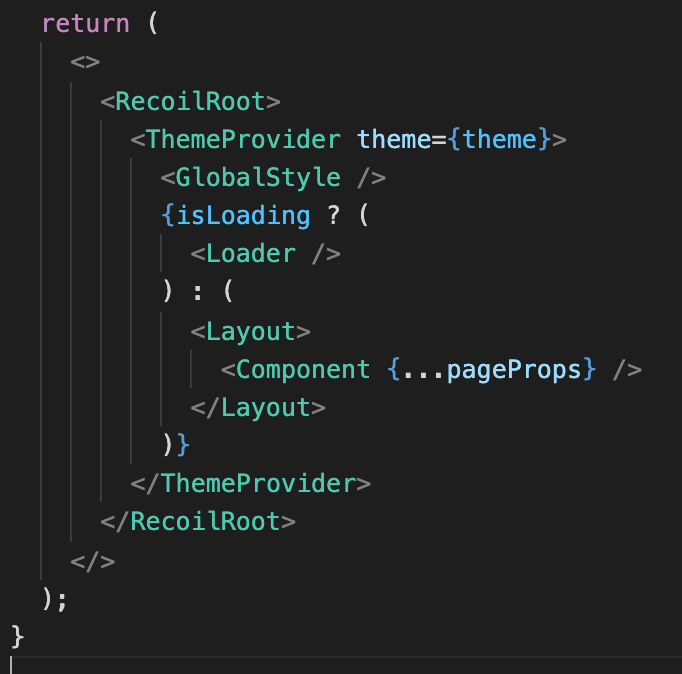
3) src > styles
styles 관련 파일들은 라이브러리 사용할 때 정리가 필요하며 일단은 그대로 냅둡니다.
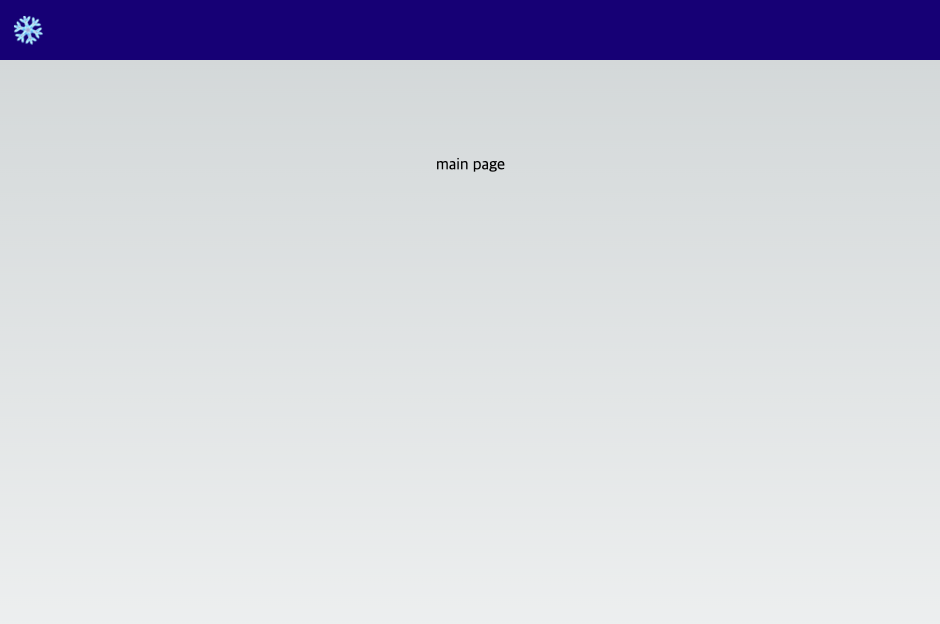
반응형
'Web > React & Next.js' 카테고리의 다른 글
Next.js 에서 Next/Image를 사용하여 이미지 처리하기 (0) | 2023.02.16 |
---|---|
Next.js | Next.js + Styled-Components 초기 세팅 (next 13) (0) | 2023.02.14 |
React | web3를 사용하여 메타마스크(Metamask) BSC 네트워크 변경 및 추가하기 (0) | 2023.01.25 |
React | axios interceptors header에 token값 설정&추가하기, 삭제하기 (0) | 2023.01.17 |
React | web3-react를 사용하여 메타마스크(Metamask) 서명 요청하기 (0) | 2023.01.17 |