Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 함수
- use Client
- nextjs contact us
- 사이트맵
- JavaScript
- pass인증
- swiper
- web3-react
- 빈도수세기
- nextjs
- 전체 너비로 css
- robots.txt
- react
- App Router
- 카카오지도 구현
- React 나이스 신원인증
- react swiper
- Next.js 나이스 본인인증
- 구글 메일보내기
- github
- 카카오맵 api
- next15
- 프로그래머스
- 메일 보내기 react
- 다중포인터
- CSS
- 프론트 본인인증
- Til
- nextjs 메일보내기
- 알고리즘
Archives
- Today
- Total
YEV.log
알고리즘 개념 00 | 알고리즘 문제에 나오는 개념 정리 본문
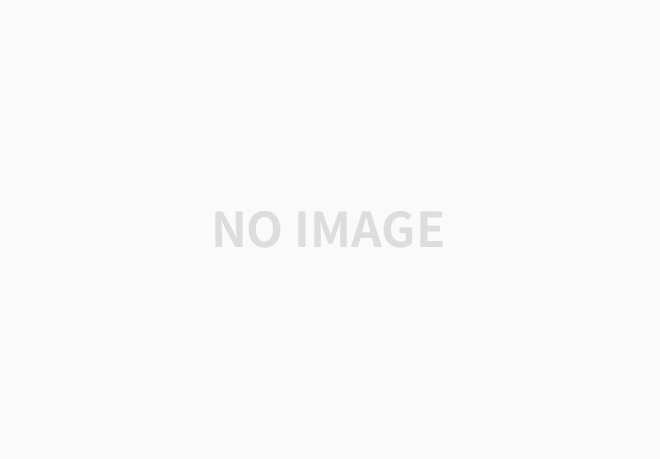
데이터/변수의 데이터 타입/자료형 알아내기
String → Number 으로, Number → String으로 데이터 타입 변환하기
동일한 문자 n번 만큼 반복하기
루트값과 제곱값 구하는 방법
value값이 정수 인지 아닌지 판단 (결과 boolean)
문자열을 일정한 구분자로 잘라서 배열로 저장
배열의 요소를 오름차순으로 정리/ 순서 뒤집기
데이터/변수의 데이터 타입/자료형 알아내기
기본형식 typeof DATA
console.log(typeof 42);
// expected output: "number"
console.log(typeof 'blubber');
// expected output: "string"
console.log(typeof true);
// expected output: "boolean"
console.log(typeof undeclaredVariable);
// expected output: "undefined"
String → Number 으로, Number → String으로 데이터 타입 변환하기
기본형식 string.repeat(count)
//String → Number
parseInt(문자); //문자를 정수형 숫자로 변환해줌
parseFloat(문자); //문자를 실수형 숫자로 변환해줌
Number(문자); //문자를 정수&실수형 숫자로 변환해줌
//Number → String
String(숫자); //숫자를 문자로 변환해줌
숫자.toString(진법); //숫자를 문자로 변환해줌 - 변환하면서 진법을 바꿀 수 있음
숫자.toFixed(소수자리수); //숫자를 문자로 변환해줌 - 실수형의 소수점 자리를 지정할 수 있음
숫자 + "문자열"; //숫자와 문자열을 한 문자열로 더해줌
동일한 문자 n번 만큼 반복하기
기본형식 string.repeat(count)
console.log("*".repeat(4)); // ****
루트값과 제곱값 구하는 방법
기본형식 Math.sqrt(n) Math.pow(n,제곱승)
//루트 값
Math.sqrt(4); //2
Math.sqrt(100); // 10
//제곱 값
Math.pow(2, 2); //4
Math.pow(10, 3); // 1000
value값이 정수 인지 아닌지 판단 (결과 boolean)
기본형식 Number.isInteger(n)
Number.isInteger(0); // true
Number.isInteger(1); // true
Number.isInteger(-100000); // true
Number.isInteger(0.1); // false
문자열/숫자를 일정한 구분자로 잘라서 배열로 저장
기본형식 string.split(구분자)
const text = 'Hello, world!';
const splitResult = text.split(','); // ["Hello", " world!"]
const splitResultByLimit0 = text.split(',', 0); // []
const splitResultByLimit1 = text.split(',', 1); // ["Hello"]
const splitResultByLimit2 = text.split(',', 2); // ["Hello", " world!"]
const num = 15;
const splitResult = String(num).split(''); // ["1", "5"]
배열의 요소를 오름차순으로 정리/ 순서 뒤집기
기본형식 arr.sort( ) arr.reverse( )
var arr = [1,4,5,3,6];
arr.sort(); //[6,5,4,3,1]
arr.reverse(); //[1,3,4,5,6]
배열의 요소 모두를 한 문자열로 출력
기본형식 arr.join('')
var arr = [1,4,5,3,6];
arr = arr.join('');
console.log(arr); // 14536
console.log(typeof arr); //string
반응형